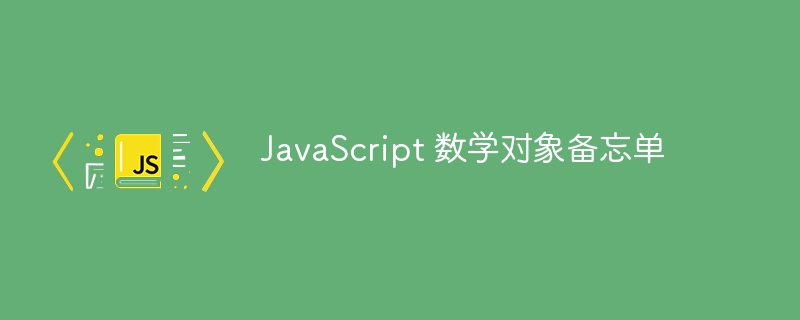
JavaScript 中的 math 对象提供了一组用于执行数学任务的属性和方法。这是 math 对象的综合备忘单。
属性
math 对象有一组常量:
Property |
description |
value (approx.) |
math.e |
euler’s number |
2.718 |
math.ln2 |
natural logarithm of 2 |
0.693 |
math.ln10 |
natural logarithm of 10 |
2.302 |
math.log2e |
base 2 logarithm of math.e |
1.442 |
math.log10e |
base 10 logarithm of math.e |
0.434 |
math.pi |
ratio of a circle’s circumference to its diameter |
3.14159 |
math.sqrt1_2 |
square root of 1/2 |
0.707 |
math.sqrt2 |
square root of 2 |
1.414 |
方法
1.舍入方法
method |
description |
example |
math.round(x) |
rounds to the nearest integer |
math.round(4.5) → 5 |
math.floor(x) |
rounds down to the nearest integer |
math.floor(4.7) → 4 |
math.ceil(x) |
rounds up to the nearest Integer |
math.ceil(4.1) → 5 |
math.trunc(x) |
removes the decimal part (truncates) |
math.trunc(4.9) → 4 |
2.随机数生成
method |
description |
example |
math.random() |
generates a random number between 0 and 1 (exclusive) |
math.random() → 0.53 |
custom random int generator |
random integer between min and max |
math.floor(math.random() * (max – min 1)) min |
3.算术方法
method |
description |
example |
math.abs(x) |
absolute value |
math.abs(-7) → 7 |
math.pow(x, y) |
raises x to the power of y |
math.pow(2, 3) → 8 |
math.sqrt(x) |
square root of x |
math.sqrt(16) → 4 |
math.cbrt(x) |
cube root of x |
math.cbrt(27) → 3 |
math.hypot(…values) |
square root of the sum of squares of arguments |
math.hypot(3, 4) → 5 |
4.指数和对数方法
method |
description |
example |
math.exp(x) |
e^x |
math.exp(1) → 2.718 |
math.log(x) |
natural logarithm (ln(x)) |
math.log(10) → 2.302 |
math.log2(x) |
base 2 logarithm of x |
math.log2(8) → 3 |
math.log10(x) |
base 10 logarithm of x |
math.log10(100) → 2 |
method |
description |
example |
math.sin(x) |
sine of x (x in radians) |
math.sin(math.pi / 2) → 1 |
math.cos(x) |
cosine of x (x in radians) |
math.cos(0) → 1 |
math.tan(x) |
tangent of x (x in radians) |
math.tan(math.pi / 4) → 1 |
math.asin(x) |
arcsine of x (returns radians) |
math.asin(1) → 1.57 |
math.acos(x) |
arccosine of x |
math.acos(1) → 0 |
math.atan(x) |
arctangent of x |
math.atan(1) → 0.785 |
math.atan2(y, x) |
arctangent of y / x |
math.atan2(1, 1) → 0.785 |
6.最小值、最大值和钳位
method |
description |
example |
math.max(…values) |
returns the largest value |
math.max(5, 10, 15) → 15 |
math.min(…values) |
returns the smallest value |
math.min(5, 10, 15) → 5 |
custom clamping |
restrict a value to a range |
math.min(math.max(x, min), max) |
7.其他方法
method |
description |
example |
math.sign(x) |
returns 1, -1, or 0 based on sign of x |
math.sign(-10) → -1 |
math.fround(x) |
nearest 32-bit floating-point number |
math.fround(5.5) → 5.5 |
math.clz32(x) |
counts leading zero bits in 32-bit binary |
math.clz32(1) → 31 |
示例
1 到 100 之间的随机整数
const randomint = math.floor(math.random() * 100) + 1; console.log(randomint);
计算圆面积
const radius = 5; const area = math.pi * math.pow(radius, 2); console.log(area); // 78.54
将度数转换为弧度
const degrees = 90; const radians = degrees * (math.pi / 180); console.log(radians); // 1.57
找到数组中最大的数字
const nums = [5, 3, 9, 1]; console.log(math.max(...nums)); // 9
数学对象的扩展用例
math 对象有许多实际应用。以下列出了常见场景和示例,以说明如何有效使用它。
1.随机化
生成一个范围内的随机整数
function getrandomint(min, max) { return math.floor(math.random() * (max - min + 1)) + min; } console.log(getrandomint(1, 10)); // random number between 1 and 10
打乱数组
function shufflearray(arr) { return arr.sort(() => math.random() - 0.5); } console.log(shufflearray([1, 2, 3, 4, 5])); // shuffled array
模拟掷骰子
function rolldice() { return math.floor(math.random() * 6) + 1; // random number between 1 and 6 } console.log(rolldice());
2.几何和形状
计算圆的面积
const radius = 5; const area = math.pi * math.pow(radius, 2); console.log(area); // 78.54
计算三角形的斜边
const a = 3, b = 4; const hypotenuse = math.hypot(a, b); console.log(hypotenuse); // 5
将度数转换为弧度
function degreestoradians(degrees) { return degrees * (math.pi / 180); } console.log(degreestoradians(90)); // 1.57
3.金融与商业
复利公式
function compoundinterest(principal, rate, time, n) { return principal * math.pow((1 + rate / n), n * time); } console.log(compoundinterest(1000, 0.05, 10, 12)); // $1647.01
四舍五入货币值
const amount = 19.56789; const rounded = math.round(amount * 100) / 100; // round to 2 decimal places console.log(rounded); // 19.57
计算折扣
function calculatediscount(price, discount) { return math.floor(price * (1 - discount / 100)); } console.log(calculatediscount(200, 15)); // $170
4.游戏和动画
模拟抛硬币
function cointoss() { return math.random() < 0.5 ? 'heads' : 'tails'; } console.log(cointoss());
平滑动画的缓动函数
function easeoutquad(t) { return t * (2 - t); // simple easing function } console.log(easeoutquad(0.5)); // 0.75
二维网格中的随机生成坐标
function randomcoordinates(gridsize) { const x = math.floor(math.random() * gridsize); const y = math.floor(math.random() * gridsize); return { x, y }; } console.log(randomcoordinates(10)); // e.g., {x: 7, y: 2}
求数组中的最大值和最小值
const scores = [85, 90, 78, 92, 88]; console.log(math.max(...scores)); // 92 console.log(math.min(...scores)); // 78
标准化数据
function normalize(value, min, max) { return (value - min) / (max - min); } console.log(normalize(75, 0, 100)); // 0.75
6.物理与工程
计算自由落体后的速度
const gravity = 9.8; // m/s^2 const time = 3; // seconds const velocity = gravity * time; console.log(velocity); // 29.4 m/s
钟摆周期
function pendulumperiod(length) { return 2 * math.pi * math.sqrt(length / 9.8); } console.log(pendulumperiod(1)); // 2.006 seconds
7.数字操纵
将数字限制在某个范围内
function clamp(value, min, max) { return math.min(math.max(value, min), max); } console.log(clamp(15, 10, 20)); // 15 console.log(clamp(5, 10, 20)); // 10
将负数转换为正数
console.log(math.abs(-42)); // 42
查找数字的整数部分
console.log(math.trunc(4.9)); // 4 console.log(math.trunc(-4.9)); // -4
8.解决问题
检查一个数字是否是 2 的幂
function ispoweroftwo(n) { return math.log2(n) % 1 === 0; } console.log(ispoweroftwo(8)); // true console.log(ispoweroftwo(10)); // false
生成斐波那契数
function fibonacci(n) { const phi = (1 + math.sqrt(5)) / 2; return math.round((math.pow(phi, n) - math.pow(-phi, -n)) / math.sqrt(5)); } console.log(fibonacci(10)); // 55
9.杂项
生成随机颜色 (rgb)
function getrandomcolor() { const r = math.floor(math.random() * 256); const g = math.floor(math.random() * 256); const b = math.floor(math.random() * 256); return `rgb(${r}, ${g}, ${b})`; } console.log(getrandomcolor()); // e.g., rgb(123, 45, 67)
根据出生日期计算年龄
function calculateAge(birthYear) { const currentYear = new Date().getFullYear(); return currentYear - birthYear; } console.log(calculateAge(1990)); // e.g., 34